一开始使用Aspose.words
来给word添加水印,尝试后发现会破坏原文件的格式,故放弃。
经过一系列资料的查找后,确认使用spire.doc for java
来实现本功能。
maven添加依赖
根据官方文档引入依赖
1 2 3 4 5 6 7 8 9 10 11 12 13
| <repositories> <repository> <id>com.e-iceblue</id> <url>http://repo.e-iceblue.cn/repository/maven-public/</url> </repository> </repositories> <dependencies> <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.doc.free</artifactId> <version>3.9.0</version> </dependency> </dependencies>
|
程序核心代码
参考官方demo的基础上,对水印的间距数量进行了优化,使多个水印能够均匀分布在一个页面上。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78
| public class SpireDocUtil {
public static void addWatermark(String srcFileName, String targetFileName, String watermarkText) { Document doc = new Document(); doc.loadFromFile(srcFileName);
Section pageSection = doc.getSections().get(0); float top = pageSection.getPageSetup().getMargins().getTop(); float right = pageSection.getPageSetup().getMargins().getRight(); float bottom = pageSection.getPageSetup().getMargins().getBottom(); float left = pageSection.getPageSetup().getMargins().getLeft(); double width = pageSection.getPageSetup().getPageSize().getWidth(); double height = pageSection.getPageSetup().getPageSize().getHeight();
ShapeObject shape = new ShapeObject(doc, ShapeType.Text_Plain_Text); int shapeWidth = 100; int shapeHeight = 12; shape.setWidth(shapeWidth); shape.setHeight(shapeHeight); double radian = 2 * Math.PI / 360 * 45; shape.setRotation(-45); shape.getWordArt().setFontFamily("SimSun"); shape.getWordArt().setText(watermarkText); shape.setFillColor(Color.lightGray); shape.setStrokeColor(Color.lightGray);
int colNum = 4; int rowNum = 6; double shapeRealWidth = Math.cos(radian) * (shapeWidth + shapeHeight); double shapeRealHeight = Math.sin(radian) * (shapeWidth + shapeHeight); double xGap = (width - shapeRealWidth * colNum) / (colNum - 1) + shapeRealWidth; double yGap = (height - shapeRealHeight * rowNum) / (rowNum - 1) + shapeRealHeight; double startX = -left - (shapeWidth - shapeRealWidth) / 2; double startY = -Math.cos(radian) * shapeHeight;
Section section; HeaderFooter header; for (int n = 0; n < doc.getSections().getCount(); n++) { section = doc.getSections().get(n); header = section.getHeadersFooters().getHeader(); Paragraph paragraph;
if (header.getParagraphs().getCount() > 0) { paragraph = header.getParagraphs().get(0); } else { paragraph = header.addParagraph(); } for (int i = 0; i < colNum; i++) { for (int j = 0; j < rowNum; j++) { shape = (ShapeObject) shape.deepClone(); shape.setHorizontalPosition((float) (startX + xGap * i)); shape.setVerticalPosition((float) (startY + yGap * j)); paragraph.getChildObjects().add(shape); } } } doc.saveToFile(targetFileName, FileFormat.Docx_2013); } }
|
最终效果
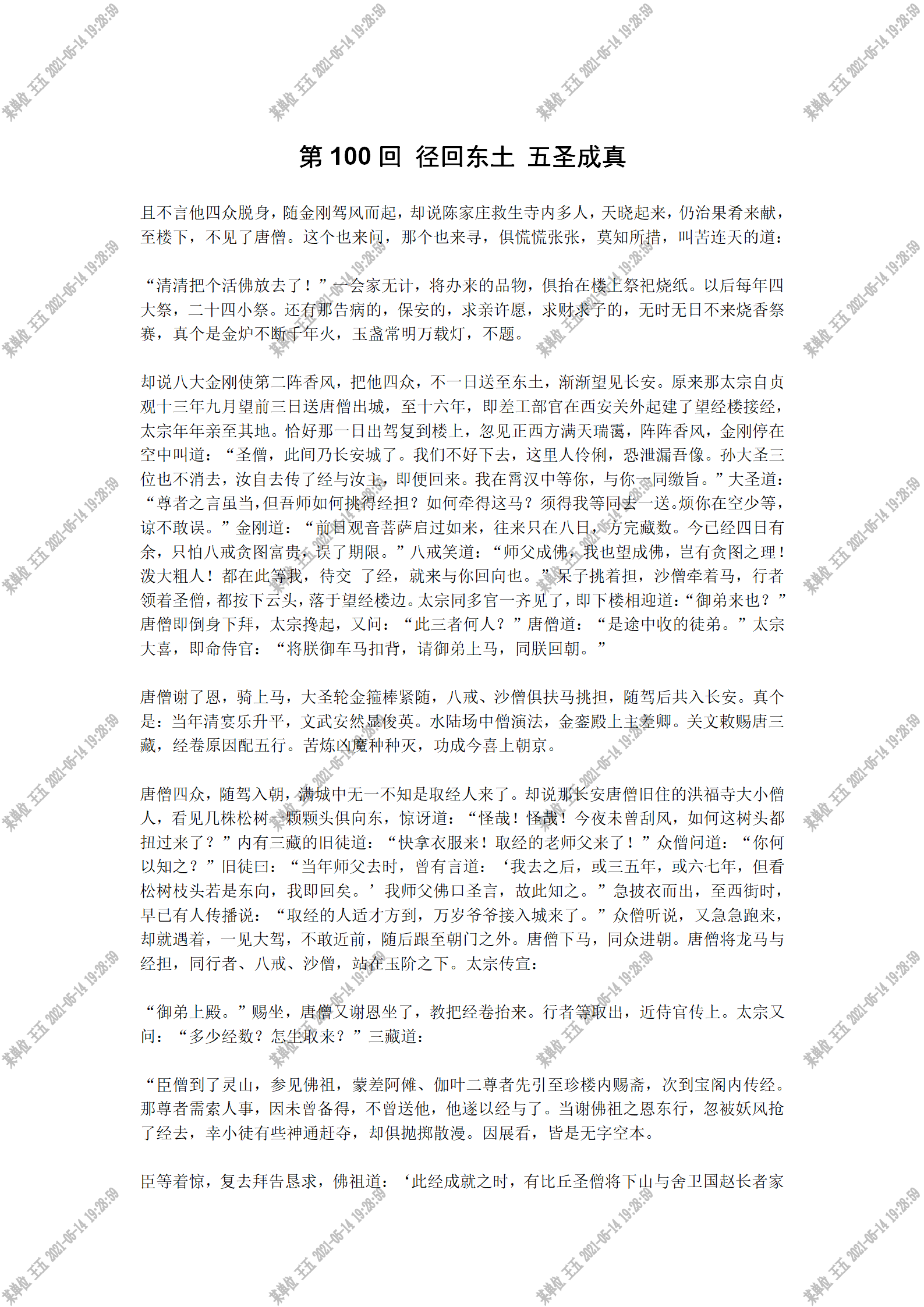